When you take photos with a digital camera or a mobile phone these devices add a lot of meta information directly to the file.
This exif data in JPG and TIFF files can be a privacy issue, which I pointed out in my TEDx talk some time ago. The photo not only shows what you want the world to see, but also when and where it was taken. You can remove the extra information in your browser if you want to. However, this extra information can also be a great thing to use in your products.
You can access EXIF information in many different ways.
* On the command line using exiftool .
* In JavaScript using Jacob Seidelin's exif.js or Mike Kovarik's more modern exifr.
* Using the in-built functions in PHP or the more detailed PEL package which also allows to write data.
* In Python using the exif package which also does read and write.
What data can you get from an image?
Take the following photo:
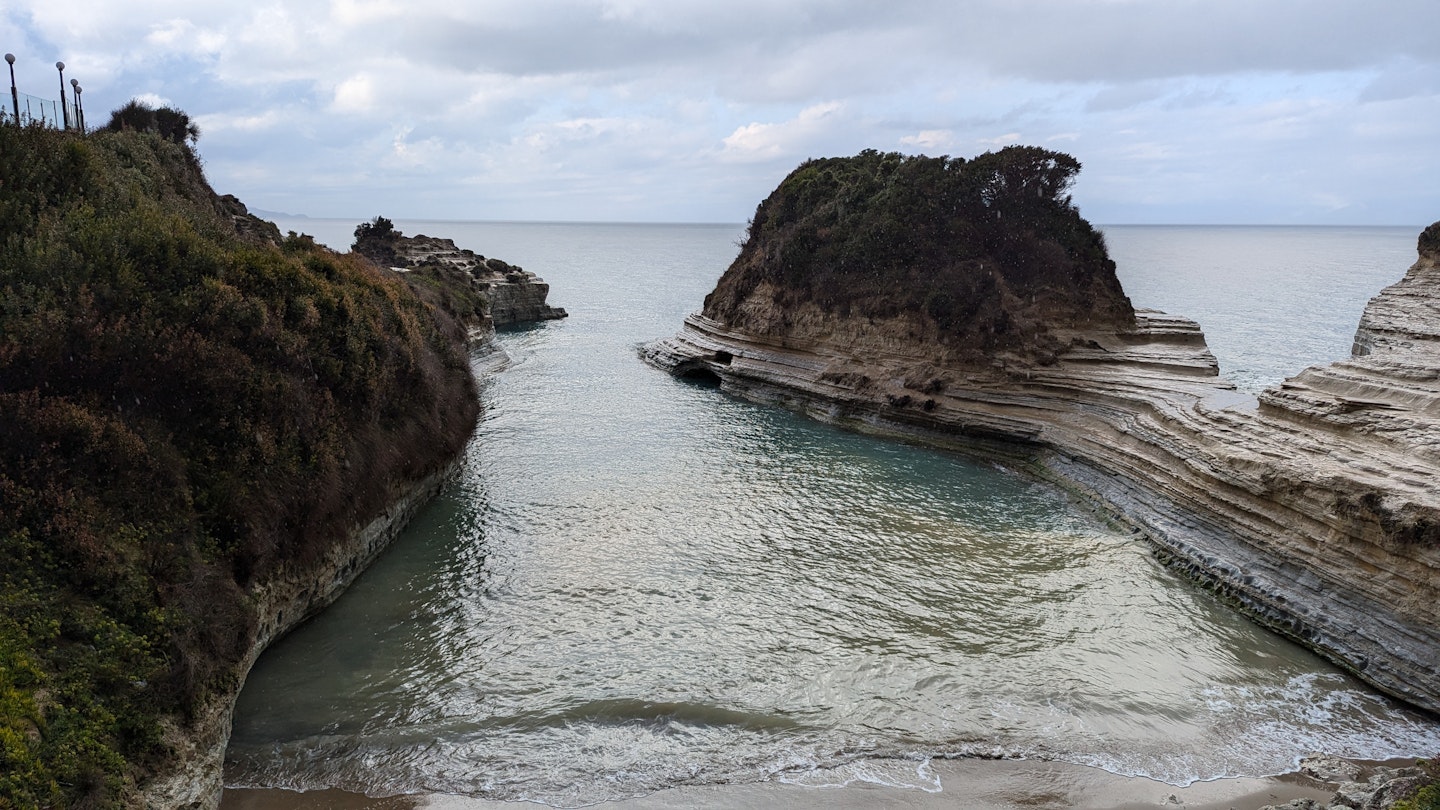
If you use any of the solutions above, you can get the following information from the image. For example, in PHP you can do a:
This results in:
What can you do with data stored by your phone?
This is a ton of information to play with. The classic is "sing the GPS data to show images on a map . If you click on the different thumbnails, you can see the map moving to where the photo was taken.
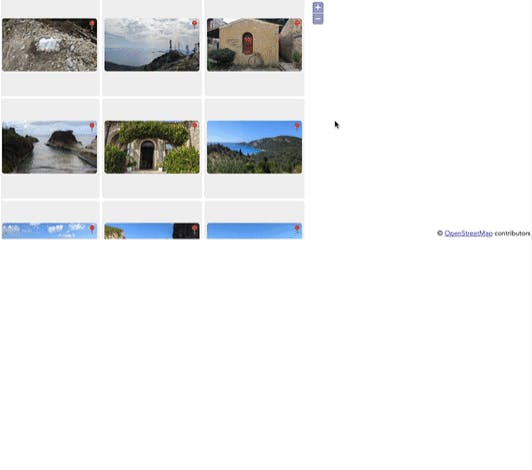
To get this information, all you need to do is read the data and then convert it.
One thing not often used though is reading the altitude information. This allows you, for example, to show the images on the height they were taken.
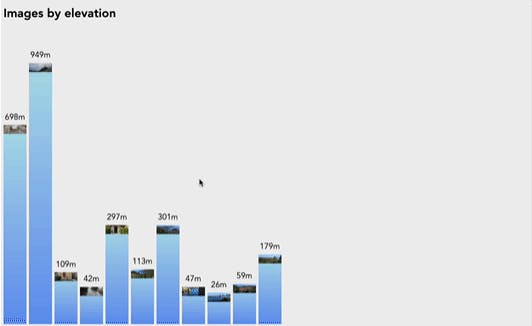
As the data is returned in a format like "4200/100" you need to do some parsing. This one creates an array of all images with the image name and its height in meters.
Depending on the device used to take the photo there might be even more information, like the angle the camera was in and if the image is portrait or landscape.
Using the embedded thumbnail information to improve web site performance
One interesting use case is to use the embedded thumbnail information to avoid having to create thumbnails. If you check the gallery or the height example and you keep developer tools open, you can see that whilst the images are all a few megabytes, the page only reads a few hundred kilobytes.
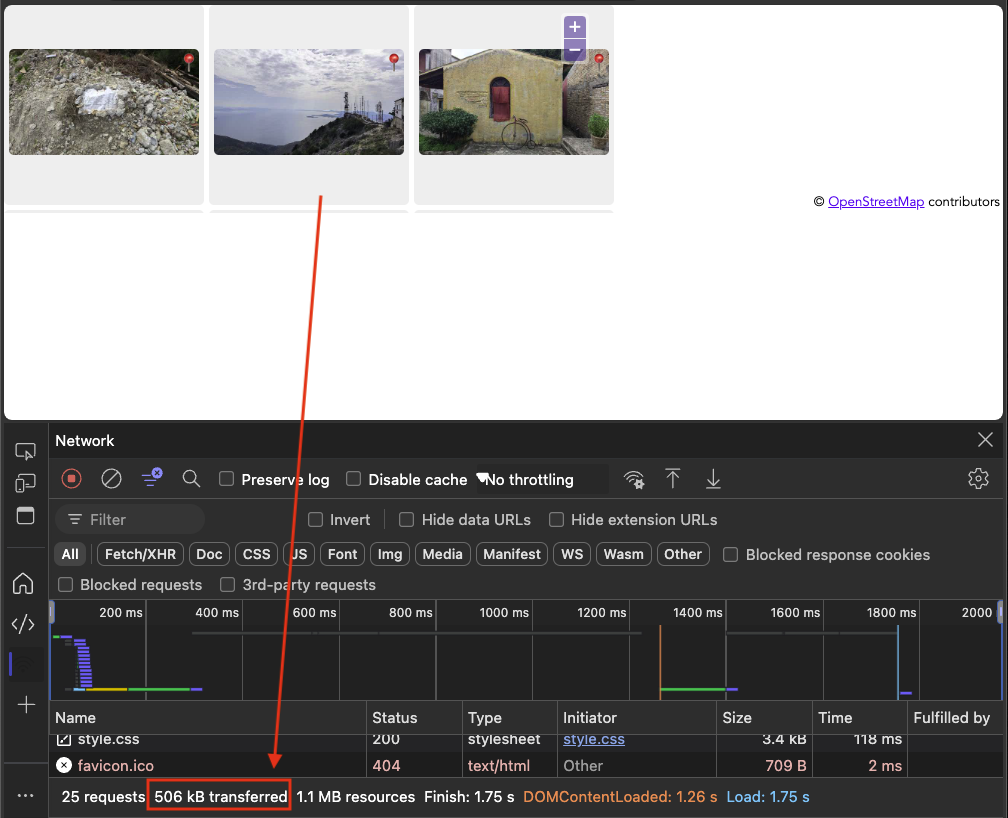
This is because we don't load the images, or created thumbnails from them, but I use the exif thumbnail information in the JPG file instead:
Originally, this thumbnail was meant to be displayed on screens built into digital cameras to have a faster preview. We can, however, also use this to preview the image without having to load it in full. In PHP this is the exif_thumbnail() method.
This will not load the whole JPG file, but only as much as it needs to get the thumbnail. This is not news. Flickr, for example, used this in the early 2000s to show you a thumbnail of the image immediately when you dragged it into the browser and then started to upload the rest. However, I have not seen it used lately. Maybe because it is too easy to create images on the cloud.
Playing with Exif is fun. And why not - after all, our phones and cameras add this information anyway.