We recently released a JSON-focused edition of the Dev Digest, honouring its creator, Douglas Crockford, and what has become one of the most commonly used formats for storing and sharing data. It turns out, developers really like JSON, so we thought we would share some of our favourite tips and tricks for working with it.
Before we get started, you can find the video-version of this article on our Watch page, and find all of the code included in this article in the GitHub repo.
Safe Parsing
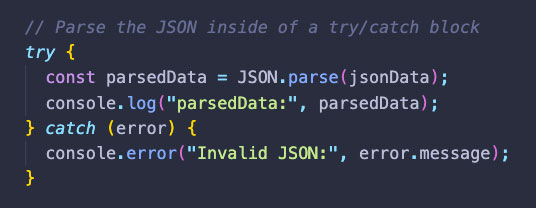
To kick things off, let's look at a simple way to safely parse JSON. We start by wrapping our JSON.parse
in a try/catch block to gracefully handle any pesky errors. Of course, we could forego this if we're absolutely certain the data is valid JSON, but it's better to be safe than sorry, right? So, let's wrap it in the try/catch, and then we can easily log errors to the console, if any arise.
Validation

Next, let's take this a step further. Let's create a simple function to check whether JSON is valid; you'll notice it contains our try/catch block, and returns a boolean confirming whether or not the JSON is valid.
This function helps ensure you're only working with legitimate JSON data, and again, makes it easy to find and understand errors if they occur.
Pretty Printing
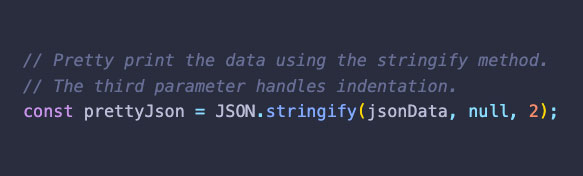
Beautifying your JSON isn't just about making it look pretty - it makes it readable too. To handle this, all you need to do is pass a number to the third parameter of the stringify method (pertaining the number of 'spaces' an indentation is made up of).
The Replacer
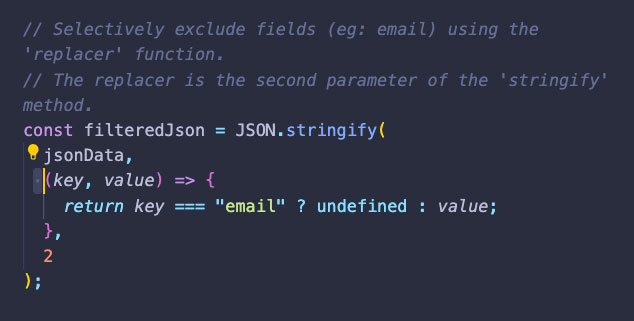
Excluding sensitive data like emails or passwords? Selectively omit fields using the replacer function, which is the second parameter of JSON.stringify.
The Reviver

The reviver works similarly, but with a key difference: the reviver allows you to transform the data at the same as parsing it. In this example, you see that I transform 'date' strings into date objects with a simple function passed in to the second parameter of JSON.parse. In this example, I target a specific object within my JSON array, targeting the date object to check it has in fact been converted to a date.
Deep Cloning
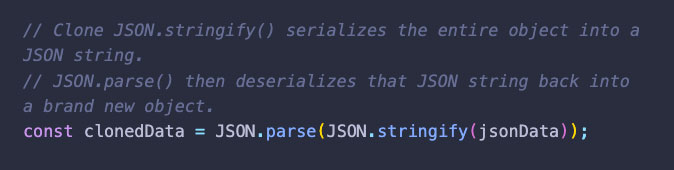
In order to avoid unexpected changes to your data, you can deep clone your JSON in order to manipulate the cloned object rather than the original. To do this, we use a simple trick where we we stringify our JSON, only to parse it once again (creating a completely new object).
In the example, we update one of the values of this new object before console.logging the values of the original object as well as the new one. This shows that we can update the cloned object while leaving the original unaffected.
Creating Query Strings
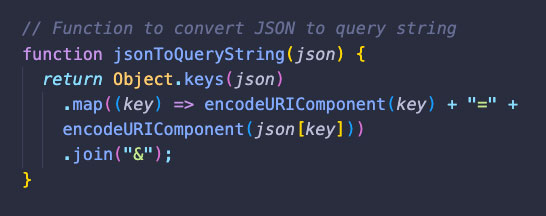
Transform your JSON data into a query string, making it easier to pass to the server or use elsewhere in your application, with a simple function. For this step, I use a simple JSON object containing a name, age and city for a fictional person.
Inside the function, we use Object.keys(params)
to get an array of all the keys in the object. In this example, this returns ["name", "age", "city"]
.
We use .map()
to go through each key and build a key-value pair string. During this step, the key and its corresponding value are both URL-encoded using encodeURIComponent()
. This ensures that special characters like spaces are safe for URLs.
The array of key-value pairs is then combined into a single string using .join('&')
. This joins each element with an '&'
symbol, forming a proper query string that is ready to use!
Dynamic JSON Creation
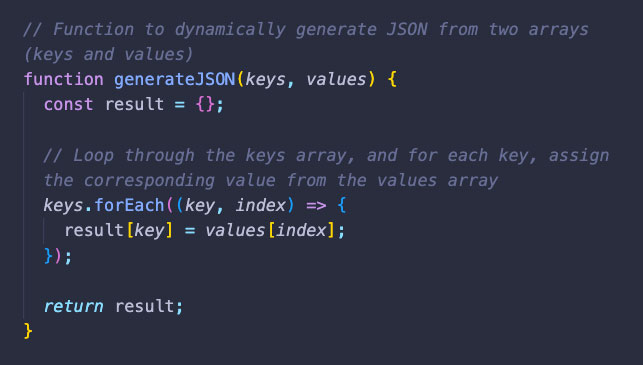
Sometimes you might receive data in separate locations, and need to use these data sources as JSON. To do this, we're going to create a function called generateJSON
.
The generateJSON
function takes two arrays, one for keys and one for values. It initializes an empty object called result
. Then, it uses forEach()
to loop through the keys
array, assigning each key a value from the values
array using the same index. The result is an object where each key from the keys
array is paired with its corresponding value from the values
array. Finally, the function returns this object.
Merging Objects
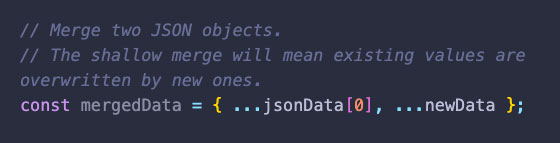
Like some of the others tips in this article, this one is not exclusive to JSON, but it's certainly useful for when you're working with it.
Merge JSON objects smoothly using the spread operator (shown below):
Here you'll notice that old data will be overwritten by new data, if any of the keys match.
Extracting Data
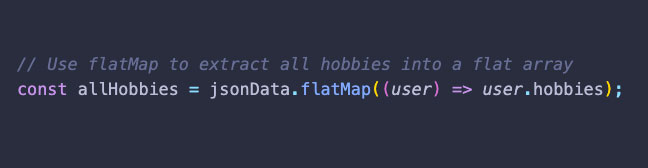
This one is a personal favourite of mine. The flapMap method essentially combines the map method with the flap method, returning a new array formed by applying a given callback function to each element of the array, and then flattening the result by one level.
The benefit of this method is that we can avoid iterating over nested arrays, making code that is difficult to read and decipher. Instead, we can just apply the flatMap method to our JSON object, targeting the nested array we want to extract from, and voilà!
Conclusion
We hope you found this article useful (or video if you watched along), and that you've picked up a few new ways of working with JSON that you can take advantage of in your own projects or at work.
You can, of course, find the code for this project on this GitHub repo, and you can submit a pull request if you would like to add your own.
Until next time... happy coding!